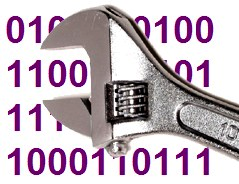
Image via Wikipedia
In any PHP or non PHP web application you should always check the data entered by the client to make sure that everything is good and there won’t be any errors if you use the data that you have received.You can do this using different methods, you can use long if and switch statements or you could simply use Filters.
In this Part of our PHP Training course we will look at Filtering the Data.
Filters are part of PHP language that will allow you to validate or Sanitize the data that your application has received from a source that you can not completely trust(and If you want your application to be secure always use them).
As I said before there are different kinds of Filtering here are the main two or you can read more about them here:
- Validating:
Validating filters as there name suggests will validate the data for you they can tell you if your data is one of the following or not, It will return a true or false and have a strict Data format that it will check against:
- Boolean ~ this will return TRUE for the following values otherwise will return FALSE “true”,”1″,”on” and “yes”.
- Valid Email Address ~ check to see if the data is a valid email address
- Valid Float ~ check to see if the data is a valid float
- Valid Integer ~ check to see if the data is a valid integer(counts +0 and -0 as a float not integer)
- Valid IP Address ~ checks to see if the data is a valid IPv4 or IPv6 IP address
- Validate it using regular expression ~ checks the data against regular expression.
- Valid URL(ASCII URLs only) ~ check the data to make sure that it is a valid URL.
- Sanitizing:
Sanitizing Filters will remove the unwanted data from the data provided they:
- Don’t have any Data format
- Always return the data(after removing the unwanted parts)
- Remove the characters in the data that are not wanted
You can add more options and flexibility to your filter using Options and Flags, Each Filter has its own options and flags and you can find the list of Options and Flags for Validating Filters and Sanitising Filters using the following link(I could list them here but it would be just repeating what is already there so just take it from the source):
To Filter Data in PHP you can use the following PHP function:
filter_var(variable, filter, options) – Filters a single variable with a specified filter
The options is an array of different option available for each filter and also if the filter accepts and you want to define a flag, you can add them in the array using the ‘flag’ field so your array would be like:
$options_array = array(
“options”=>array(“min_range”=>0,”max_range”=>256, “flags”=>FILTER_FLAG_ALLOW_HEX)
);
The above array will work for integer filter and here is an example:
<?php
$month=14;$options_array = array(
“options”=>array(“min_range”=>1,”max_range”=>12)
);if(!filter_var($month, FILTER_VALIDATE_INT, $options_array)){
echo “$month is not a valid month”;
}else{
echo “$month is a valid month”;
}
?>
There are different functions in PHP that allows for Filtering the data but they are out of scope of this Basic PHP Tutorial.
You can Validate an array using the same or different filters at the same time using filter_var_array() or Validate the input data directly using filter_input() or get an array as an input and filter them using filter_input_array() you can find more about each using the link provided.
